React Native Tech Stack 2025: The Ultimate Guide#
The mobile development landscape in 2025 has evolved significantly, and React Native stands at the forefront of this evolution. As the framework becomes more robust, performant, and stable, choosing the right tools and packages for your project has never been more crucial. This comprehensive guide will help you navigate the vast ecosystem of React Native development and make informed decisions for your next project.
Core Development Setup#
When starting a new React Native project in 2025, there are two fundamental choices that form the foundation of your development setup:
Expo Framework#
Expo has emerged as the de-facto standard for React Native development in 2025. Here's why you should choose Expo for your next project:
- Official Recognition: Expo is now the recommended framework in the React Native documentation
- Production Ready: Major companies have adopted Expo for their production applications
- Developer Experience: Provides a comprehensive development environment out of the box
- Framework Benefits: Avoiding the need to build your own framework from scratch
- Migration Friendly: Even legacy projects using the React Native CLI can gradually migrate to Expo
TypeScript Integration#
TypeScript has become an essential tool in modern React Native development. Here's why you should embrace it:
- Enhanced Developer Experience: Get real-time feedback from your IDE
- Bug Prevention: Catch type-related errors before they reach production
- Maintainability: Makes your codebase more maintainable and self-documenting
- Practical Usage: Focus on practical type implementations rather than complex type patterns
- IDE Support: Excellent integration with modern development environments
While it's possible to develop React Native applications without TypeScript, the benefits it brings to your development workflow are invaluable. The type system helps prevent common errors and provides better code documentation, making it easier for teams to collaborate and maintain code over time.
Navigation Solutions#
When it comes to navigation in React Native, there are two main contenders in 2025:
Expo Router vs React Navigation#
Both solutions are powerful, but they serve different needs:
Expo Router
Expo Router has become the preferred choice for modern React Native applications. Here's why:
- File-based Routing: Intuitive and familiar for web developers
- Cross-platform Focus: Excellent support for iOS, Android, and web
- Advanced Features:
- API routes support
- RC (Remote Components) support
- Built-in layouts system
- Future-proof: Regular updates and new features from the Expo team
React Navigation
React Navigation remains a solid choice, especially if:
- You're focusing solely on native mobile platforms
- You prefer a more traditional screen-based approach
- You need more granular control over navigation
Enhanced Navigation Components#
For tab-based navigation, consider using React Native Bottom Tabs, which provides:
- Native bottom tabs implementation
- Smooth animations on both platforms
- Better performance than custom implementations
UI and Styling Solutions#
The UI and styling landscape in React Native has evolved to offer multiple approaches, each with its own strengths:
Modern Styling Solutions#
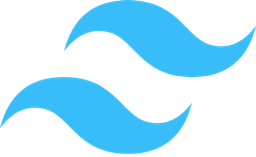
- NativeWind NativeWind brings Tailwind CSS to React Native:
- Familiar Tailwind syntax
- Growing ecosystem with NativeWind UI components
- Strong community adoption
- UniStyles UniStyles offers:
- Familiar StyleSheet-like API
- Enhanced functionality
- C++ bindings for optimal performance
- Version 3.0 with new features
- Tamagui Tamagui provides:
- Cross-platform styling solution
- Optimized compiler
- Built-in component library
- Strong performance optimizations
Component Libraries#
While traditional component libraries like React Native Paper and React Native Elements still have their place, modern development tends to favor more flexible solutions:
- NativeWind UI: Pre-built components using NativeWind
- React Native Reusables: Chakra-inspired components for React Native
- Tamagui Components: Cross-platform optimized components
Choosing the Right Solution#
Your choice should depend on:
- Project requirements
- Team expertise
- Performance needs
- Cross-platform requirements
- Design system flexibility
For most modern projects, NativeWind offers an excellent balance of features, familiarity, and flexibility. However, UniStyles provides a more native-feeling approach, while Tamagui excels in cross-platform scenarios with its optimization features.
Animation Libraries#
Animation is crucial for creating engaging mobile experiences. Here are the top choices for 2025:
React Native Reanimated#
React Native Reanimated remains the gold standard for animations:
- High-performance native animations
- Declarative API
- Excellent TypeScript support
- Rich ecosystem and community
Moti#
Moti offers a simpler approach to animations:
- Built on top of Reanimated
- Easier learning curve
- Preset animations
- Great for quick implementations
State Management#
Modern React Native apps require different state management solutions for different use cases:
Server State Management#
TanStack Query (formerly React Query) is the clear winner for managing server state:
- Powerful data synchronization
- Built-in caching
- Automatic background updates
- Optimistic updates
- Request deduplication
Alternative: Redux Toolkit Query
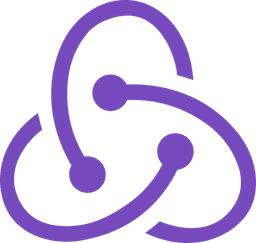
If your project is already using Redux for client state management, Redux Toolkit Query (RTK Query) is an excellent alternative:
- Tight integration with Redux ecosystem
- Code generation for API endpoints
- Automatic cache management
- Built-in TypeScript support
- Familiar Redux patterns
The choice between TanStack Query and RTK Query often depends on your existing architecture:
- Choose TanStack Query for new projects or when you prefer a standalone solution
- Use RTK Query when you're already invested in the Redux ecosystem
Application State Management#
Several solutions exist for managing client-side state:
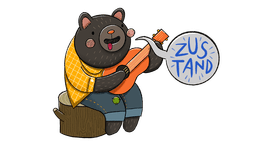
- Zustand Zustand is the preferred choice for most applications:
- Simple and lightweight
- Familiar store concept
- Great TypeScript support
- Strong community adoption
- Legend State Legend State for local-first applications:
- Built-in sync capabilities
- Supabase integration
- Offline-first approach
- Context API React's built-in Context API might be sufficient for:
- Simple state requirements
- Small to medium applications
- Component-level state sharing
Data Storage Solutions#
Database ORM Solutions#
Drizzle ORM
Drizzle ORM has emerged as the modern, lightweight alternative to traditional ORMs in 2025:
-
Key Features:
- TypeScript-first approach
- SQL-like query builder
- Zero abstractions over SQL
- Significantly smaller bundle size
- Better performance than traditional ORMs
- Built-in schema migrations
- Native Edge runtime support
-
Benefits:
- Type-safe database queries
- Minimal runtime overhead
- Direct SQL-like syntax
- Easy integration with SQLite and Postgres
- Excellent developer experience
- Strong community growth
While Prisma has been widely used in previous years, Drizzle represents the future of database interactions with its lightweight approach and modern architecture. It's particularly well-suited for React Native applications due to its:
- Smaller bundle size impact
- Better performance characteristics
- Simpler mental model
- Native Edge compatibility
Migration from Prisma
If you're coming from Prisma, Drizzle offers several advantages:
- More predictable query generation
- Faster query execution
- No need for additional CLI tools
- Direct SQL-like syntax that's easier to debug
- Better suited for edge environments
Supabase#
Supabase has become the go-to backend solution for React Native applications in 2025:
-
Core Features:
- PostgreSQL Database with real-time capabilities
- Built-in authentication and user management
- Edge Functions for serverless computing
- Storage solution for files and media
- Auto-generated TypeScript types
- Official React Native SDK
-
Key Benefits:
- Excellent offline-first capabilities
- Row Level Security (RLS) for data protection
- WebSocket connections for real-time features
- Competitive pricing with generous free tier
- Strong TypeScript integration
SQLite with Expo#
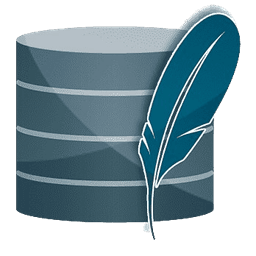
Expo SQLite provides a reliable and easy-to-use local database solution:
-
Key Features:
- Built into Expo SDK
- Full SQLite API access
- Async/Sync API support
- Transaction support
- TypeScript support
- Key-value storage API
-
Use Cases:
- Offline-first applications
- Local data caching
- Complex local queries
- Structured data storage
- Migration from existing SQLite databases
-
Integration Options:
- SQL query execution
- Prepared statements
- Database migrations
- Key-value storage mode
- React hooks integration with SQLiteProvider
Choose SQLite when you need:
- Complete control over your data structure
- Complex local queries
- Familiar SQL syntax
- Proven reliability
- Small app size
Essential Developer Tools#
Testing Tools#
- Maestro Maestro for UI testing:
- Easy setup
- Reliable UI testing
- Great developer experience
- React Native Testing Library React Native Testing Library:
- Industry standard for unit testing
- Comprehensive documentation
- Large community support
Monitoring and Debugging#
- Sentry Sentry for production monitoring:
- Real-time error tracking
- Performance monitoring
- Crash reporting
- Debug information
- Reactotron Reactotron for development debugging:
- API monitoring
- State management debugging
- Performance tracking
CI/CD Solutions#
Expo EAS (Expo Application Services) EAS provides:
- Cloud builds
- Automatic app submission
- Over-the-air updates
- Workflow automation
- Seamless integration with Expo projects
These tools form a robust development environment that can significantly improve your React Native development workflow. The combination of these solutions provides a comprehensive setup for building, testing, and deploying professional React Native applications.
Essential Utility Packages#
These packages are considered must-haves for specific functionalities in React Native development:
Storage and Performance#
- React Native MMKV MMKV for high-performance storage:
- Fastest key-value storage available
- Native implementation
- Perfect alternative to AsyncStorage
- Minimal setup required
- FlashList FlashList for optimized list rendering:
- Drop-in replacement for FlatList
- Significantly better performance
- Similar API to FlatList
- Built-in recycling system
Form Management#
React Hook Form React Hook Form with Zod for form handling:
- Performant form validation
- Type-safe with Zod integration
- Minimal re-renders
- Great React Native support
Image Management#
Expo Image Expo Image for optimized image handling:
- Built-in caching
- Memory management
- Progressive loading
- Cross-platform optimization
UI Interactions#
- Zego Zego for advanced UI interactions:
- Beautiful dropdown menus
- Context menus
- Force touch overlays
- Native feel
- React Native Gesture Handler Gesture Handler for native-powered gestures:
- Pan gestures
- Long press
- Rotation
- Fling gestures
- Built-in Expo support
Authentication and Monetization#
- Clerk Clerk for comprehensive auth management:
- Complete user management
- Social provider integration
- Security best practices
- Easy implementation
- RevenueCat RevenueCat for in-app purchases:
- Cross-platform purchase management
- Easy product sync
- Analytics and insights
- Simplified implementation
Camera Integration#
React Native Vision Camera Vision Camera for advanced camera features:
- High-performance camera access
- Photo and video capture
- QR code scanning
- Barcode recognition
- Native implementation
Conclusion#
The React Native ecosystem in 2025 is more mature and robust than ever. While the UI landscape might continue to evolve, the core tools and utilities have stabilized, providing a solid foundation for building professional mobile applications. This tech stack represents the best-in-class solutions for various development needs, backed by strong communities and proven in production environments.
The combination of these tools enables developers to create high-performance, maintainable, and feature-rich applications while maintaining excellent developer experience. As the React Native ecosystem continues to grow, these recommendations will help you make informed decisions for your next mobile project.